Overview
U-Schedule is a desktop calendar application for university students written for the project for the module CS2103 Software Engineering. The user interacts with it using a CLI, and it has a GUI created with JavaFX for user feedback. It is written in Java, and has about 10 kLoC.
Summary of contributions
-
Major enhancement: added the ToDoList feature
-
What it does: It allows users to record the tasks with different priorities that need to be completed and delete if completed.
-
Justification: This feature improves the product significantly because in addition to those events that occur at specific times, users also need to record tasks that do not have a specific time. These tasks can be assignments, projects or some simple plans. And they can do tasks according to priority.
-
Highlights: This enhancement uses a different model from calendar events and it need re-construct logic, storage and ui conponents.
-
-
Minor enhancement:
-
Add a showDescriptionCommand to show description of task with a popup window.
-
Make priorities displayed in different colors.
-
-
Code contributed: https://nus-cs2103-ay1819s1.github.io/cs2103-dashboard/#=undefined&search=sleepysanjinli
-
Other contributions:
-
Project management:
-
Set up some issues.
-
Managing milestones.
-
-
Enhancements to existing features:
-
Implement GUI for todo lisk (Pull requests https://github.com/CS2103-AY1819S1-T10-1/main/pull/64)
-
-
Documentation:
-
Did cosmetic tweaks to existing contents of the User Guide and Developer Guide: https://github.com/CS2103-AY1819S1-T10-1/main/pull/120
-
-
Community:
-
PRs reviewed (with non-trivial review comments): https://github.com/CS2103-AY1819S1-T10-1/main/pull/130
-
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Showing description: show todo
Shows description of the specified todo item from the todo list.
Format: show todo INDEX
Examples:
-
list todo
show todo 1
Shows description of the 1st todo item in the todo list.
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
ToDoList feature
Current implementation
Model for ToDoList feature:
The ToDoList Model ModelToDo
allows the ToDoList
to store and display ToDoListEvents
.
ToDoListEvent
stores the relevant information for each event in the toDoList, such as the title, description and priority.
AddToDoCommandParser
and DeleteToDoCommandParser
functionaly have been created and allow user to add and delete ToDoListEvent
objects.
Prefixes t/, d/ and p/ respectively allow user to input Title
, Description
and Priority
for each todolist event.
Given below is an example usage scenario for adding a todolist event, and how the todolist model behaves at each step.
Step 1: The user lauches the application for the first time. The ToDoList
is initialised to be empty.
Step 2: The user excutes add todo t/CS3230 Assignment1 d/Dynamic Programming P/H
.
Step 3: The AddToDoCommandParser
parsers out the strings for the title, description and priority.
Step 4: Then, ParserUtil
checks that the input strings are valid, and uinitialises the Title
, Description
and Priority
. It also checks that the Priority
iniput is a valid priority.
Step 5: Following that, a new ToDoListEvent
is initialised and will be displayed in the list of todo list events in the GUI.
Given below is an example usage scenario for deleting a todolist event, and how the todolist model behaves at each step.
Step 1: Make sure the todo list is not empty.
Step 2: The user executes delete todo [index of event]
while [index of event]
is the index number for a corresponding todolist event` displayed in ToDoList
GUI.
Step 3: The DeleteToDoCommandParser
parsers out the strings for the index.
Step 4: Then, ParserUtil
checks that the input index is valid.
Step 5: Following that, the corresponding ToDoListEvent
will be deleted and not displayed on th GUI.
The following sequece diagram shows how the add todo operation works:
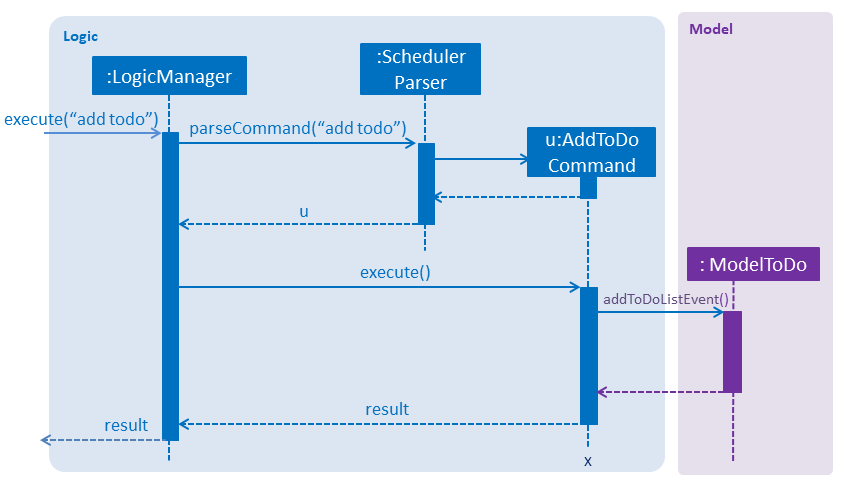
The delete todo does the similar operation.It calls ModelToDo#deleteToDoListEvent
.
ShowDescriptionParser
functionaly have been created and allow user to show description of ToDoListEvent
objects.
Given below is an example usage scenario for showing description of a todolist event, and how the todolist model behaves at each step.
Step 1: Make sure the todo list is not empty.
Step 2: The user executes show todo [index of event]
while [index of event]
is the index number for a corresponding todolist event` displayed in ToDoList
GUI.
Step 3: The ShowDescriptionCommandParser
parsers out the strings for the index.
Step 4: Then, ParserUtil
checks that the input index is valid.
Step 5: Following that, the corresponding description of ToDoListEvent
will be showed and displayed by DescriptionDisplay
.
The following sequece diagram shows how the show todo operation works:
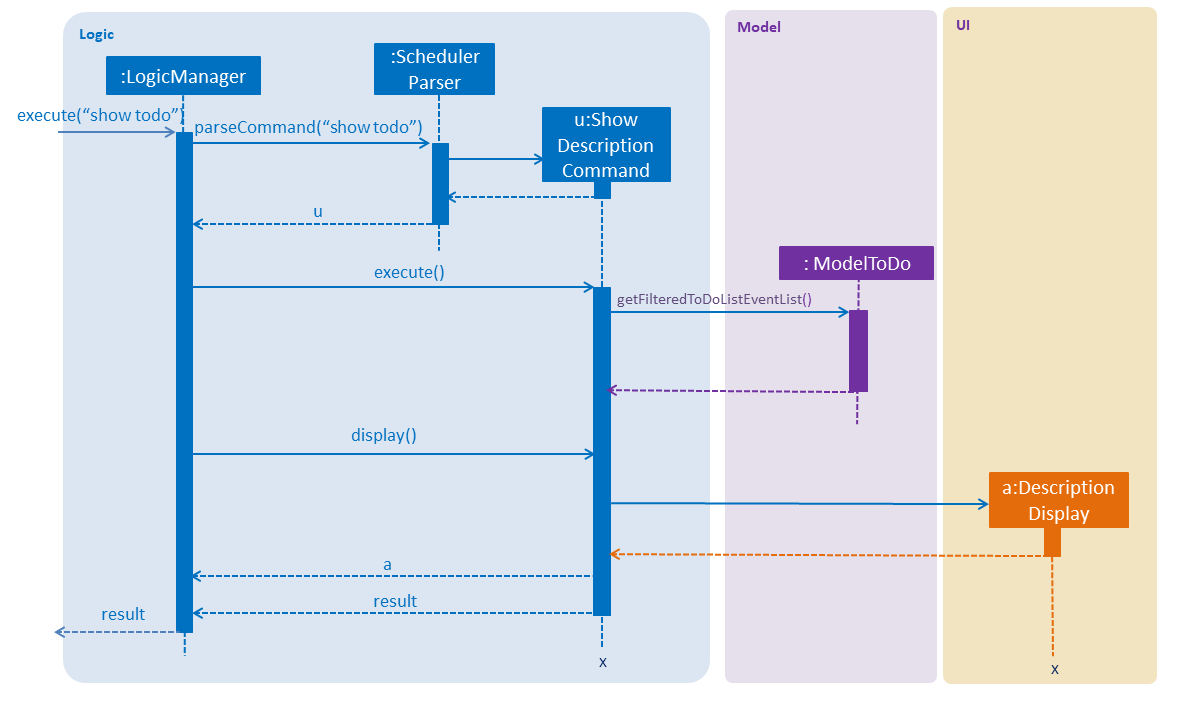
Implementation for list todo
is similar with list event
.
Step 1: The user executes list todo
.
Step 2: The ListToDoCommand
will be executed.
Step 3: The tab will change to display todo list panel.
Logic for ToDoList feature:
-
Logic#getFilteredToDoListEventList
— Returns an unmodifiable view of the filtered list ofToDoListEvent
. -
LogicManager#ModelToDo
— Model forToDoListEvent
.
commands
-
Command
— Abstract class for executing todo list. -
Command#isToDoCommand
— Judge the command is todo command or not. -
Command#excute(ModelToDo, CommandHistory)
— Execute todo command. -
AddToDoCommand
/DeleteToDoCommand
— ExtendsCommand
foradd todo
/delete todo
functionality. -
ListToDoCommand
— ExtendsCommand
forlist todo
functionality. -
ShowDescriptionCommand
— ExtendsCommand
forshow todo
functionality.
parsers
-
SchedulerParser#parserCommand
— Add additionl parses user input into command for execution.
Storage for ToDoList feature:
-
ToDoListStorage
— Represents a storage forToDoList
. -
XmlAdaptedToDoListEvent
— JAXB-friendly version of theToDoListEvent
. -
XmlSerializableToDoList
— An ImmutableToDoList
that is serializable to XML format -
XmlToDoListStorage
— A class to accessToDoList
data stored as an xml file on the hard disk. -
Storage
— Add Extension toToDoListStorage
. -
StorageManager
— AddToDoListStorage
component.
GUI for ToDoList feature:
The ToDoList GUI contains two parts:
-
TaskListPanel
— Panel containing the list ofToDoListEvent
. -
ToDoListEventCard
— An UI component that displays information of aToDoListEvent
.
In ToDoListEventCards
, there are four components:
-
ToDoListEventCard#CheckBox
— For check completed task. -
ToDoListEventCard#Label(id)
— For display index of correspondingToDoListEvent
. -
ToDoListEventCard#Label(title)
— For display title of correspondingToDoListEvent
. -
ToDoListEventCard#Label(priority)
— For display priority of correspondingToDoListEvent
. And different priorities will be displayed in different colors.
The description popup GUI is developed by DescriptionDisplay
, there are two components:
-
DescriptionDisplay#TextArea(description)
— For display description of correspondingToDoListEvent
. -
DescriptionDisplay#Label(id)
— For display index of correspondingToDoListEvent
.
Design Considerations
Aspect: How to implement ToDoList Model
-
Alternative 1 (current choice): Re-construct a new model for ToDoList.
-
Pros: Will not influence current functionalities for Calendar part.
-
Cons: Time cost (Need to re-construct a lot of classes).
-
-
Alternative 2: Modify current calendar model to support both events.
-
Pros: Need less classes to re-construct.
-
Cons: May cause some trouble in curent Calendar model.
-
Aspect: How to implement ToDoList Logic
-
Alternative 1 (current choice): Modify the current
Logic
andLogicManager
.-
Pros: Can be easily implemented and can use the current parser.
-
-
Alternative 2: Re-construct a new
LogicToDo
forToDoList
.-
Pros: Will not influence current Scheduler Logic.
-
Cons: Difficult to implement in
MainApp
and other combined classes (need anotherLogicToDo
object).
-
Aspect: How to implement ToDoList Storage
-
Alternative 1 (current choice): Modify the curernt
Storage
andStorageManager
.-
Pros: Can be easily implemented.
-
Cons: Need to extends one more
ToDoListStorage
.
-
-
Alternative 2: Re-construct a new
StorageToDo
forToDoList
.-
Pros: Will not influence current
Storage
. -
Cons: Difficult to implement in
MainApp
and other combined classes. Cause redundant work.
-
Aspect: How to implement ToDoList UI
-
Alternative 1 (current choice): Extend current GUI and left side become ToDoList GUI.
-
Pros: Will be synchronous for
ToDoList
andCalendar
. -
Cons: Need to modify current GUI.
-
-
Alternative 2: Totally seperate to be 2 GUI (including two
CommandBox
).-
Pros: Don’t need to change current GUI.
-
Cons: Need to consruct a lot parts in GUI.
-
And modify diagrams in Design parts, including Architecture, UI Component, Logic Component and Storage Component.